Build an IoT Smart Farm Using Raspberry Pi and Actian Zen
Johnson Varughese
September 26, 2024
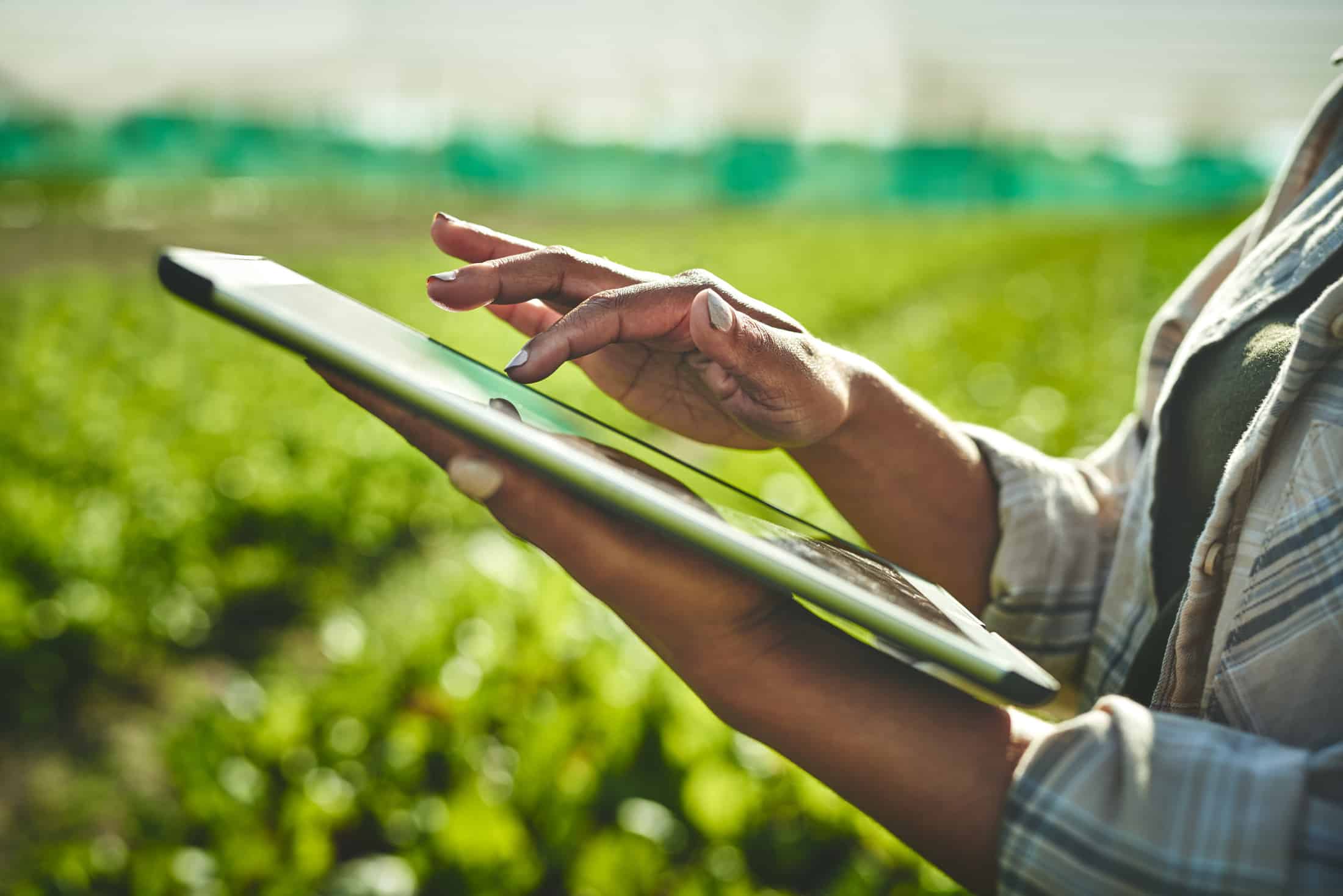
Technology is changing every industry, and agriculture is no exception. The Internet of Things (IoT) and edge computing provide powerful tools to make traditional farming practices more efficient, sustainable, and data-driven. One affordable and versatile platform that can form the basis for such a smart agriculture system is the Raspberry Pi.
In this blog post, we will build a smart agriculture system using IoT devices to monitor soil moisture, temperature, and humidity levels across a farm. The goal is to optimize irrigation and ensure optimal growing conditions for crops. We’ll use a Raspberry Pi running Raspbian OS, Actian Zen Edge for database management, Zen Enterprise to handle the detected anomalies on the remote server database, and Python with the Zen ODBC interface for data handling. Additionally, we’ll leverage AWS SNS (Simple Notification Service) to send alerts for detected anomalies in real-time for immediate action.
Prerequisites
Before we start, ensure you have the following:
- A Raspberry Pi running Raspbian OS.
- Python installed on your Raspberry Pi.
- Actian Zen Edge database installed.
- PyODBC library installed.
- AWS SNS set up with an appropriate topic and access credentials.
Step 1: Setting Up the Raspberry Pi
First, update your Raspberry Pi and install the necessary libraries:
sudo apt-get update sudo apt-get upgrade sudo apt-get install python3-pip pip3 install pyodbc boto3
Step 2: Install Actian Zen Edge
Follow the instructions on the Actian Zen Edge download page to download and install Actian Zen Edge on your Raspberry Pi.
Step 3: Create Tables in the Database
We need to create tables to store sensor data and anomalies. Connect to your Actian Zen Edge database and create the following table:
CREATE TABLE sensor_data ( id identity PRIMARY KEY, timestamp DATETIME, soil_moisture FLOAT, temperature FLOAT, humidity FLOAT );
Install Zen Enterprise, connect to the central database, and create the following table:
CREATE TABLE anomalies ( id identity PRIMARY KEY , timestamp DATETIME, soil_moisture FLOAT, temperature FLOAT, humidity FLOAT, description longvarchar );
Step 4: Define the Python Script
Now, let’s write the Python script to handle sensor data insertion, anomaly detection, and alerting via AWS SNS.
Anomaly Detection Logic
Define a function to check for anomalies based on predefined thresholds:
def check_for_anomalies(data): threshold = {'soil_moisture': 30.0, 'temperature': 35.0, 'humidity': 70.0} anomalies = [] if data['soil_moisture'] < threshold['soil_moisture']: anomalies.append('Low soil moisture detected') if data['temperature'] > threshold['temperature']: anomalies.append('High temperature detected') if data['humidity'] > threshold['humidity']: anomalies.append('High humidity detected') return anomalies
Insert Sensor Data
Define a function to insert sensor data into the database:
import pyodbc def insert_sensor_data(data): conn = pyodbc.connect('Driver={Pervasive ODBC Interface};servername=localhost;Port=1583;serverdsn=demodata;') cursor = conn.cursor() cursor.execute("INSERT INTO sensor_data (timestamp, soil_moisture, temperature, humidity) VALUES (?, ?, ?, ?)", (data['timestamp'], data['soil_moisture'], data['temperature'], data['humidity'])) conn.commit() cursor.close() conn.close()
Send Anomalies to the Remote Database
Define a function to send detected anomalies to the database:
def send_anomalies_to_server(anomaly_data): conn = pyodbc.connect('Driver={Pervasive ODBC Interface};servername=<remote server>;Port=1583;serverdsn=demodata;') cursor = conn.cursor() cursor.execute("INSERT INTO anomalies (timestamp, soil_moisture, temperature, humidity, description) VALUES (?, ?, ?, ?, ?)", (anomaly_data['timestamp'], anomaly_data['soil_moisture'], anomaly_data['temperature'], anomaly_data['humidity'], anomaly_data['description'])) conn.commit() cursor.close() conn.close()
Send Alerts Using AWS SNS
Define a function to send alerts using AWS SNS:
def send_alert(message): sns_client = boto3.client('sns', aws_access_key_id='Your key ID', aws_secret_access_key ='Your Access key’, region_name='your-region') topic_arn = 'arn:aws:sns:your-region:your-account-id:your-topic-name' response = sns_client.publish( TopicArn=topic_arn, Message=message, Subject='Anomaly Alert' ) return response
Replace your-region, your-account-id, and your-topic-name with your actual AWS SNS topic details.
Step 5: Generate Sensor Data
Define a function to simulate real-world sensor data:
import random import datetime def generate_sensor_data(): return { 'timestamp': datetime.datetime.now().strftime('%Y-%m-%d %H:%M:%S'), 'soil_moisture': random.uniform(20.0, 40.0), 'temperature': random.uniform(15.0, 45.0), 'humidity': random.uniform(30.0, 80.0) }
Step 6: Main Function to Simulate Data Collection and Processing
Finally, put everything together in a main function:
def main(): for _ in range(100): sensor_data = generate_sensor_data() insert_sensor_data(sensor_data) anomalies = check_for_anomalies(sensor_data) if anomalies: anomaly_data = { 'timestamp': sensor_data['timestamp'], 'soil_moisture': sensor_data['soil_moisture'], 'temperature': sensor_data['temperature'], 'humidity': sensor_data['humidity'], 'description': ', '.join(anomalies) } send_anomalies_to_server(anomaly_data) send_alert(anomaly_data['description']) if __name__ == "__main__": main()
Conclusion
And there you have it! By following these steps, you’ve successfully set up a basic smart agriculture system on a Raspberry Pi using Actian Zen Edge and Python. This system, which monitors soil moisture, temperature, and humidity levels, detects anomalies, stores data in databases, and sends notifications via AWS SNS, is a scalable solution for optimizing irrigation and ensuring optimal growing conditions for crops. Now, it’s your turn to apply this knowledge and contribute to the future of smart agriculture.
Remember to replace placeholders with your actual AWS SNS topic details and database connection details. Happy farming!
Subscribe to the Actian Blog
Subscribe to Actian’s blog to get data insights delivered right to you.
- Stay in the know – Get the latest in data analytics pushed directly to your inbox
- Never miss a post – You’ll receive automatic email updates to let you know when new posts are live
- It’s all up to you – Change your delivery preferences to suit your needs